Using the JavaScript Fetch API asynchronously
Published on 2020-10-25 • Modified on 2020-10-25
In this snippet, we will see how to use the JavaScript Fetch API asynchronously. Open your JavaScript console and click on the "fetch data" button. As you can see, the message at the end of the snippet118()
function is displayed before the JSON is received. For more clarity, I made a subfunction fetchAsynch()
which is declared as async
, and that is responsible for returning the promise associated with the fetch
call.
/**
* I am using a JavaScript module to isolate the code of each snippet.
* In fact it's a Vue.js mixin. Take the code called by the mounted()
* or the snippetXX() function.
*/
export default {
data: {
apiEndpoint: '/stats'
},
methods: {
snippet118 () {
console.log('snippet118() call.')
this.fetchAsynch().then(json => {
console.log('Received JSON!!')
console.log(json)
}).catch(error => {
console.log('An error occured sorry: ' + error)
})
console.log('snippet118() end.')
},
async fetchAsynch () {
const response = await fetch(this.apiEndpoint)
if (!response.ok) {
const message = `Error : ${response.status}`
throw new Error(message)
}
return await response.json()
}
}
}
More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
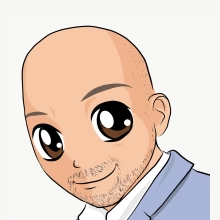