Getting the active Symfony application's environment
Published on 2020-10-10 • Modified on 2020-10-10
In this snippet, we will see how to get the active Symfony application's environment. There are two ways of doing it: by using the kernel or by getting the kernel.environment
services container's parameter. Both methods must return the same value. Note that when using the dotenv component, one could also use the $_ENV['APP_ENV']
superglobal, but it's not a good practice.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use Symfony\Component\HttpKernel\KernelInterface;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property KernelInterface $kernel
*/
trait Snippet116Trait
{
public function snippet116(): void
{
$fromKernel = $this->kernel->getEnvironment();
$fromParameters = $this->getParameter('kernel.environment');
if ($fromKernel !== $fromParameters) {
throw new \RuntimeException('Houston, We Have a Problem. 💥');
}
echo \sprintf('The current active application environment is "%s".', $fromKernel); // That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Read the doc
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet116Trait
*/
final class Snippet116Test extends KernelTestCase
{
protected function setUp(): void
{
self::bootKernel();
}
/**
* @see Snippet116Trait::snippet116
*/
public function testSnippet116(): void
{
self::assertSame(self::$kernel->getEnvironment(), self::getContainer()->getParameter('kernel.environment'));
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
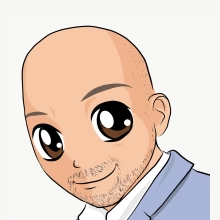