Integer type validation of a PHP variable
Published on 2024-01-19 • Modified on 2024-01-19
In a previous snippet, we saw a (ugly) hack to convert a string variable to an integer. Here, we validate with the multi-purpose filter_var()
function. If the variable is not an integer, then it returns false. The specific case for true
is something to know as it returns true
even if it's a boolean. As we can see in the output, true
is transformed to 1. It is weird as it is not the case for false
.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet287Trait
{
public function snippet287(): void
{
$tests = [
true,
false,
'0.0',
'foo',
['my' => 'array'],
555,
0,
1,
-1,
];
foreach ($tests as $test) {
$filteredVar = filter_var($test, FILTER_VALIDATE_INT);
if ($filteredVar !== false) {
echo \sprintf('%s is an integer, final var: %s'.PHP_EOL, var_export($test, true), var_export($filteredVar, true));
} else {
echo \sprintf('%s is NOT an integer'.PHP_EOL, var_export($test));
}
}
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
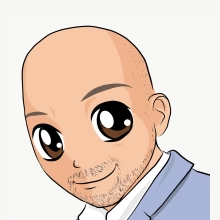