Converting a CSV file into Symfony entities
Published on 2024-07-21 • Modified on 2024-07-21
This snippet shows how to convert a CSV file into Symfony entities. Instead of manually setting each property, we can use the Symfony denormalizer. If a specific process must be done we can use a custom denormalizer.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Entity\Article;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet310Trait
{
public function snippet310(): void
{
$csvFile = \dirname(__DIR__, 3).'/data/articles.csv';
$file = new \SplFileObject($csvFile);
$file->setFlags(\SplFileObject::READ_CSV);
$idx = 0;
$columns = [];
foreach ($file as $fields) {
// get properties' names
if (++$idx === 1) {
/** @var array<int|string> $columns */
$columns = $fields;
continue;
}
/** @var array<string, string> $fields */
if (!(\count(array_filter($fields)) > 0)) { // ignore last empty line
continue;
}
$entityArray = array_combine($columns, $fields);
// prepare data before denormalization (this could be done in a custom denormalizer)
$entityArray['active'] = (bool) $entityArray['active'];
/** @var Article $entity */
$entity = $this->serializer->denormalize($entityArray, Article::class);
echo \sprintf('-- Entity %s n°%d', get_debug_type($entity), $idx).PHP_EOL;
echo \sprintf('type: %s', $entity->getType()->value).PHP_EOL;
echo \sprintf('active: %s', $entity->active() ? 'true' : 'false').PHP_EOL;
echo \sprintf('name: %s', $entity->getName()).PHP_EOL;
echo \sprintf('in language: %s', $entity->active()).PHP_EOL.PHP_EOL;
}
// That's it! š
}
}
Run this snippet More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky š¦
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges š .
Thank you for reading! And see you soon on Strangebuzz! š
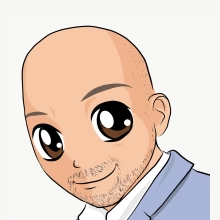