Caching data with Symfony
Published on 2022-03-13 • Modified on 2022-03-13
This snippet shows how to cache data with Symfony. This one is for me because I always forget how to do. We have to inject the CacheInterface
then use the get()
function with a closure. This is the most straightforward way. In this example, the cached content depends on the current locale. Wait five seconds and refresh the page to have a new value. Refresh the page before to verify that the cached content is correctly returned. Using a DateInterval
object is a good practice as it is more explicit than using an integer (for the number of seconds). You can also do all the job in one line as showed in the commented example.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Contracts\Cache\CacheInterface;
use Symfony\Contracts\Cache\ItemInterface;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property CacheInterface $cache
*/
trait Snippet192Trait
{
public function snippet192(Request $request): void
{
$locale = $request->getLocale();
$cached = $this->cache->get('random_cached_'.$locale, function (ItemInterface $item) use ($locale) {
$item->expiresAfter(\DateInterval::createFromDateString('5 seconds'));
echo "Generating new cache for '$locale'...".PHP_EOL;
return $locale.'/'.random_int(0, 100000).'/Strangebuzz.com';
// you can also use a one liner
// return $item->expiresAfter(\DateInterval::createFromDateString('5 seconds'))->set($locale.'/'.random_int(0, 100000).'/Strangebuzz.com')->get();
});
echo 'cache value: '.$cached;
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
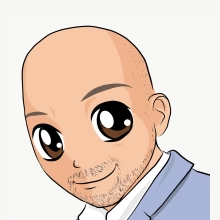