Trimming a word at the start of a string with PHP
Published on 2022-03-05 • Modified on 2022-03-05
This snippet shows how to trim a word (or string) at the start of a string with PHP or Symfony. In the following example, we can't use the str_replace
function as the strange:)
string would also be replaced. With PHP, we can use the preg_replace
function, and with Symfony, we can use the elegant trimPrefix()
function of the string component introduced in Symfony 5.4/6.0. Much more readable, right? 🙂
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use function Symfony\Component\String\u;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet191Trait
{
public function snippet191(): void
{
$string = "strange: It is strange:) isn't it?";
$prefix = 'strange:';
// With PHP
$withPhp = preg_replace('/^'.preg_quote($prefix, '/').'/', '', $string);
// With Symfony
$withSymfony = u($string)->trimPrefix($prefix);
echo 'string: '.$string.PHP_EOL.PHP_EOL;
echo 'PHP: '.$withPhp.PHP_EOL;
echo 'Symfony: '.$withSymfony;
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc More on the web
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
use function Symfony\Component\String\u;
/**
* @see Snippet191Trait
*/
final class Snippet191Test extends KernelTestCase
{
/**
* @see Snippet191Trait::snippet191
*/
public function testSnippet191(): void
{
$string = "strange: It is strange:) isn't it?";
$prefix = 'strange:';
$withPhp = preg_replace('/^'.preg_quote($prefix, '/').'/', '', $string);
$withSymfony = u($string)->trimPrefix($prefix);
self::assertSame(" It is strange:) isn't it?", $withPhp);
self::assertSame($withPhp, $withSymfony->toString());
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
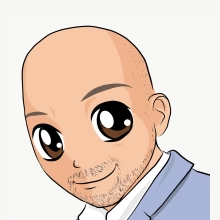