Adding attributes to HTML tags with PHP
Published on 2023-10-01 • Modified on 2023-10-01
This snippet shows how to add attributes to HTML tags with PHP. In the following example, we use the DOMDocument
library to parse some HTML, get the links and add to them a class attribute with the "pretty" value. We must use the LIBXML_HTML_NOIMPLIED LIBXML_HTML_NODEFDTD
flags, or the output will contain extra body and doctype tags.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet277Trait
{
public function snippet277(): void
{
$html = '<ul><li><a href="/foo">foo</a></li><li><a href="/bar">bar</a></li></ul>';
echo $html;
$dom = new \DOMDocument();
$dom->loadHTML($html, LIBXML_HTML_NOIMPLIED | LIBXML_HTML_NODEFDTD);
$aTags = $dom->getElementsByTagName('a');
foreach ($aTags as $aTag) {
$aTag->setAttribute('class', 'pretty');
}
echo PHP_EOL;
echo $dom->saveHTML();
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
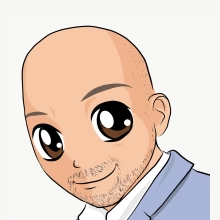