Using the PHP null coalescing assignment operator
Published on 2022-09-09 • Modified on 2022-09-09
This snippet shows how to use the PHP null coalescing assignment operator. One learns every day! I had totally forgotten about this new PHP 7.4 functionality! We all know the classic coalescing operator ??
because it significantly improved our life as of PHP 7.0. As its name indicates, the PHP null coalescing assignment operator allows to use the coalescing operator, and simultaneously assigns the result to a variable. In the following example, we give a value to the bar3
key; this key is not defined as the two random previous keys have a length of eight characters. So the value is set. But, in the last assignment, the value isn't modified as the key already exists. We use array_key_last
, and the array contains three values, so it will always return the last key foo
we set.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use Symfony\Component\String\ByteString;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet217Trait
{
public function snippet217(): void
{
$myArray = [];
$myArray[ByteString::fromRandom(8)->toString()] = 'bar1';
$myArray[ByteString::fromRandom(8)->toString()] = 'bar2';
$myArray['foo'] ??= 'bar3';
var_dump($myArray);
$myArray[array_key_last($myArray)] ??= 'not modified';
var_dump($myArray);
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc More on the web
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use PHPUnit\Framework\TestCase;
use Symfony\Component\String\ByteString;
/**
* @see Snippet217Trait
*/
final class Snippet217Test extends TestCase
{
/**
* @see Snippet217Trait::snippet217
*/
public function testSnippet217(): void
{
$myArray = [];
$myArray[ByteString::fromRandom(8)->toString()] = 'bar1';
$myArray[ByteString::fromRandom(8)->toString()] = 'bar2';
$myArray['foo'] ??= 'bar3';
self::assertSame('bar3', $myArray['foo']);
$myArray[array_key_last($myArray)] ??= 'not modified';
self::assertSame('bar3', $myArray['foo']);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
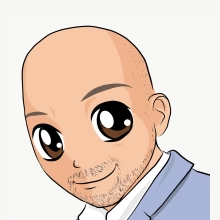