Testing if an array is a list with PHP
Published on 2022-09-10 • Modified on 2022-09-10
This snippet shows how to test if an array is a list with PHP. This function was introduced in PHP 8.1, before we had to do this manually. Check out the StackOverflow question for more details. You can now find snippets related to new PHP 8.1 functionalities with the PHP 8.1 tag. Note that PHPStan already analyses array_is_list()
calls and raises an error is the calls are useless, like in the following unit tests.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet218Trait
{
public function snippet218(): void
{
$myList = [1, 2, 4, 8];
var_dump($myList);
var_dump(array_is_list($myList)); // @phpstan-ignore-line
$myList['foo'] = 'bar'; // extra non numeric keys, the array is not a list anymore
var_dump($myList);
var_dump(array_is_list($myList)); // @phpstan-ignore-line
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use PHPUnit\Framework\TestCase;
/**
* @see Snippet218Trait
*/
final class Snippet218Test extends TestCase
{
/**
* @see Snippet218Trait::snippet218
*/
public function testSnippet218(): void
{
$myList = [1, 2, 4, 8];
self::assertTrue(array_is_list($myList)); // @phpstan-ignore-line Yes PHPStan but we want to test by ourselves.
$myList['foo'] = 'bar';
self::assertFalse(array_is_list($myList)); // @phpstan-ignore-line Same here
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
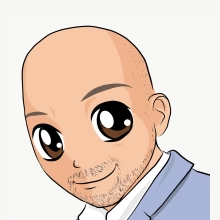