Using reduce to extract a result from a JavaScript array
Published on 2020-07-19 • Modified on 2020-07-19
In this snippet, we will see how to extract a result from a JavaScript array using the reduce
function and a callback. First, we define two callbacks; they will test if the article is a post or a snippet using an arrow function. Then we use these two "reducers" as the first parameter of the reduce
function. The initial value is, of course zero, as we do an accumulation. Open your JavaScript console to check the results.
/**
* I am using a JavaScript module to isolate the code of each snippet.
* In fact it's a Vue.js mixin. Take the code called by the mounted()
* or the snippetXX() function.
*/
export default {
methods: {
snippet103 () {
const articles = [
{ label: 'Article 1', type: 1 },
{ label: 'Snippet 1', type: 2 },
{ label: 'Snippet 2', type: 2 }
]
const isPost = (accumulator, article) => accumulator + (article.type === 1 ? 1 : 0)
const isSnippet = (accumulator, article) => accumulator + (article.type === 2 ? 1 : 0)
console.log('The articles array contains ' + articles.reduce(isPost, 0) + ' post(s).')
console.log('The articles array contains ' + articles.reduce(isSnippet, 0) + ' snippets(s).')
}
},
mounted () {
if (this.isArticle(103)) {
this.snippet103()
}
}
}
More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
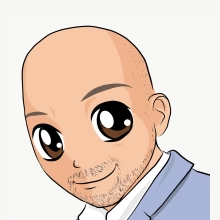