[PHP] Using array_reduce with a closure to extract a result from an array
Published on 2020-07-11 • Modified on 2020-07-11
In this snippet, we will see how to extract a result from an array using the array_reduce
PHP function and a closure. I get all the articles in the database, and then I compute two counts: the posts' count and the snippets' count. Of course, this is just for the example as it would have been better to do it with SQL.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Entity\Article;
use App\Repository\ArticleRepository;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property ArticleRepository $articleRepo
*/
trait Snippet100Trait
{
public function snippet100(): void
{
$articles = $this->articleRepo->findActive();
$postsCount = array_reduce($articles, static function ($carry, Article $article) {
return (int) $carry + ($article->isArticle() ? 1 : 0);
});
$snippetsCount = array_reduce($articles, static function ($carry, Article $article) {
return (int) $carry + ($article->isSnippet() ? 1 : 0);
});
echo 'Articles count:'.\count($articles).PHP_EOL;
echo 'Blog posts count:'.$postsCount.PHP_EOL;
echo 'Snippets count:'.$snippetsCount;
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use App\Entity\Article;
use App\Repository\ArticleRepository;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet100Trait
*/
final class Snippet100Test extends KernelTestCase
{
private ArticleRepository $articleRepo;
protected function setUp(): void
{
$this->articleRepo = self::getContainer()->get(ArticleRepository::class);
}
/**
* @see Snippet100Trait::snippet100
*/
public function testSnippet100(): void
{
$articles = $this->articleRepo->findActive();
$postsCount = (int) array_reduce($articles, static function ($carry, Article $article): int {
return (int) $carry + ($article->isArticle() ? 1 : 0);
});
$snippetsCount = (int) array_reduce($articles, static function ($carry, Article $article): int {
return (int) $carry + ($article->isSnippet() ? 1 : 0);
});
// An article is either a blog post or a snippet, the article type is mandatory
self::assertSame(\count($articles), $postsCount + $snippetsCount);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
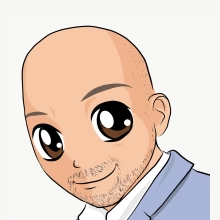