Trimming the first word of a string with PHP
Published on 2021-11-28 • Modified on 2021-12-03
In this snippet, we see how to trim the first word of a string with PHP. In the following example, we can't use a simple replace function as the "DoNotremoveMe" would be modified too, which we want to avoid. So, we can use the preg_replace
function and use the ^
special character that points out the start of a string. With the Symfony string component we can use the replaceMatches
function with the same regexp. We mustn't forget to escape the word in case it contains special regexp characters (not the case here with "removeMe").
[Edit 2021-12-03] Great news: As of Symfony 5.4, there is a new trimPrefix()
function for this purpose.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Helper\String\StringHelper;
use function Symfony\Component\String\s;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property StringHelper $stringHelper
*/
trait Snippet176Trait
{
public function snippet176(): void
{
$string = 'removeMe|meow|DoNotremoveMe|cat';
$word = 'removeMe';
$excapedWord = preg_quote($word, '/');
echo 'string: '.$string;
echo "\n";
echo 'needle: '.$word;
echo "\n\n";
echo "—— With pure PHP —————————————————————————————————————————————————\n";
$result = preg_replace("/^$excapedWord/", '', $string);
echo $result;
echo "\n";
echo "\n";
echo "—— With the Symfony string component —————————————————————————————\n";
echo s($string)->replaceMatches("/^$excapedWord/", '')->toString();
echo "\n";
echo "\n";
echo "—— Or, as of Symfony 5.4 —————————————————————————————————————————\n";
echo s($string)->trimPrefix($word);
echo "\n";
echo "\n";
echo "—— With my Symfony string component helper ———————————————————————\n";
echo $this->stringHelper->s($string)->trimStartingWord($word);
echo "\n";
// this trimStartingWord() function is just a shortcut for the previous call.
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc More on the web
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use App\Helper\String\StringHelper;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
use function Symfony\Component\String\s;
/**
* @see Snippet176Trait
*/
final class Snippet176Test extends KernelTestCase
{
private StringHelper $stringHelper;
protected function setUp(): void
{
$this->stringHelper = self::getContainer()->get(StringHelper::class);
}
/**
* @see Snippet176Trait::snippet176
*/
public function testSnippet176(): void
{
$string = 'removeMe|meow|DoNotremoveMe|cat';
$word = 'removeMe';
$escapedWord = preg_quote($word, '/');
$expected = '|meow|DoNotremoveMe|cat';
$result = preg_replace("/^$escapedWord/", '', $string);
self::assertSame($expected, $result);
$result = s($string)->replaceMatches("/^$escapedWord/", '')->toString();
self::assertSame($expected, $result);
$result = s($string)->trimPrefix($word)->toString();
self::assertSame($expected, $result);
$result = $this->stringHelper->s($string)->trimStartingWord($word);
self::assertSame($expected, $result);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
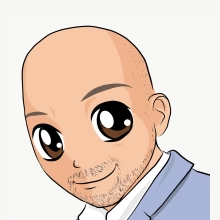