Getting the JSON request body as an array with Symfony
Published on 2021-12-04 • Modified on 2021-12-04
In this snippet, we see how to get the JSON request body as an array with Symfony. I still see projects trying to decode $request->getContent()
, but you can use the Request::toArray()
function. Note that if the content is empty or invalid, it raises a JsonException
. This function was introduced in Symfony 5.2.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use Symfony\Component\HttpFoundation\Exception\JsonException;
use Symfony\Component\HttpFoundation\Request;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet177Trait
{
public function snippet177(Request $request): void
{
// This throws an exception as we access the run snippet page with GET and
// without JSON request body.
try {
$requestAsArray = $request->toArray();
var_dump(\count($requestAsArray));
} catch (JsonException $e) {
echo $e->getMessage()."\n";
}
// Now let's create a new request with valid JSON
$manualRequest = new Request([], [], [], [], [], [], '{"foo":"bar"}');
echo "\nNew request body is not empty and valid:\n";
var_dump($manualRequest->toArray());
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc More on the web
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use PHPUnit\Framework\TestCase;
use Symfony\Component\HttpFoundation\Exception\JsonException;
use Symfony\Component\HttpFoundation\Request;
/**
* @see Snippet177Trait
*/
final class Snippet177Test extends TestCase
{
/**
* @see Snippet177Trait::snippet177
*/
public function testSnippet177(): void
{
$request = new Request([], [], [], [], [], [], '');
$this->expectException(JsonException::class);
$request->toArray();
$request = new Request([], [], [], [], [], [], '{"foo": bar}');
$requestArray = $request->toArray();
self::assertIsArray($requestArray);
self::assertSame($requestArray['foor'], 'bar');
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
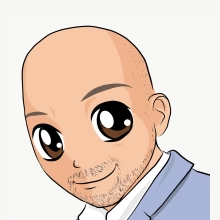