Testing a getter and a setter at the same time in a PHPUnit test
Published on 2022-10-26 • Modified on 2022-10-26
This snippet shows how to test a getter and a setter simultaneously in a PHPUnit test case. This small trick allows us to gain space and test both functions on the same line thanks to the fluent interface of the class. You can use a data provider if you don't want to duplicate the tested value.
<?php
declare(strict_types=1);
namespace App\Tests\Unit\Entity;
use App\Entity\Article;
use PHPUnit\Framework\TestCase;
final class ArticleTest extends TestCase
{
public function testArticleIcon(): void
{
self::assertNull((new Article())->getIcon()); // initial value, field is nullable
self::assertSame('icon', (new Article())->setIcon('icon')->getIcon());
}
/**
* @return iterable<array{0: string}>
*/
public function providerForArticleIconWithProvider(): iterable
{
yield ['foo'];
yield [''];
}
/**
* @dataProvider providerForArticleIconWithProvider
*/
public function testArticleIconWithProvider(string $value): void
{
self::assertSame($value, (new Article())->setIcon($value)->getIcon());
}
}
More on Stackoverflow More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
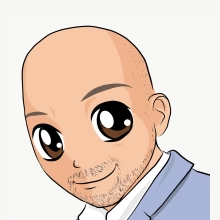