[PHP] Sorting an array by descending numerical value
Published on 2020-02-07 • Modified on 2020-02-07
In this snippet, we will see how to sort a PHP array by descending numerical value. That the type of code I sometimes forget and where I must check the documentation. I thought at first that we had to pass the SORT_NUMERIC
flag to the arsort
function but it isn't required if the values are well formatted numbers.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet76Trait
{
public function snippet76(): void
{
$myArray = [
'orange' => 3,
'lemon' => 1,
'banana' => 5,
'kiwi' => 11,
'apple' => 2,
];
print_r($myArray);
arsort($myArray);
print_r($myArray);
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet76Trait
*/
final class Snippet76Test extends KernelTestCase
{
/**
* @see Snippet76Trait::snippet76
*/
public function testSnippet76(): void
{
$myArray = [
'orange' => 3,
'lemon' => 1,
'banana' => 5,
'kiwi' => 11,
'apple' => 2,
];
arsort($myArray);
$values = array_values($myArray);
self::assertSame(11, $values[0]);
self::assertSame(1, $values[\count($myArray) - 1]);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
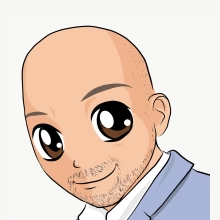