PHP 8.1: using the first-class callable syntax to create a callback
Published on 2022-10-02 • Modified on 2022-10-02
This snippet shows how to use the new first-class callable syntax introduced in PHP 8.1. Creating a callback has never been the most straightforward thing in PHP. This new feature tries to address this. Instead of using arrays and strings, we can now use functions and calls as we would typically do in the current context where the callback is required. The different thing is the usage of the ...
spread operator instead of potential arguments. It also allows static analysis to work with it. And obviously, in the following code, if the function you try to call doesn't exist, your IDE will immediately raise an error, even before the static analysis.
Haha, this is a funny note in the official documentation: The ...
is part of the syntax and not an omission. 😁
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet221Trait
{
public function snippet221(): void
{
$array = [
'a',
'bb',
'ccc',
'dddd',
];
print_r($array);
$array = array_map($this->callbackForSnippet221(...), $array);
print_r($array);
// before il was array_map('strlen',
$array = array_map(strlen(...), $array);
print_r($array);
// That's it! 😁
}
public function callbackForSnippet221(string $element): string
{
return $element.' ('.\strlen($element).')';
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
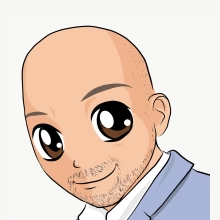