Generating a slug with the Symfony string component
Published on 2022-10-11 • Modified on 2022-10-11
This snippet shows how to generate a slug with the Symfony string component. Usually, when using the Symfony string component, we use the u()
shortcut. Not in this case, there is a dedicated class for this purpose. It's easy to use and test as it doesn't have dependencies. The most common parameter, the separator, is available as the second argument of the slug()
function. You can also get the service with the SluggerInterface
type hint. Be careful that the final string isn't in lowercase by default. You can also use the Doctrine sluggable extension for your entities, which is convenient.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use Symfony\Component\String\Slugger\AsciiSlugger;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet222Trait
{
public function snippet222(): void
{
$slugger = new AsciiSlugger(); // you can type-hint SluggerInterface to get slugger as a service
$articleTitle = 'Generating a slug with the Symfony string component';
$slug = $slugger->slug($articleTitle)->lower();
echo $slug.PHP_EOL;
$slug = $slugger->slug($articleTitle, '_')->lower();
echo $slug.PHP_EOL;
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
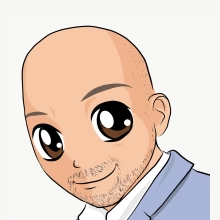