Looping on JavaScript arrays
Published on 2020-06-19 • Modified on 2020-06-19
In this snippet, we will see how to loop on JavaScript arrays. I will go straight to the point and show my preferred ways, the first without using ES6 and the two following supporting it. For full explanations, check out the StackOverflow link. The main answer is very exhaustive; the pros and cons are explained for each alternative. As always, open your JavaScript console and click on the buttons to check the results.
/**
* I am using a JavaScript module to isolate the code of each snippet.
* In fact it's a Vue.js mixin. Take the code called by the mounted()
* or the snippetXX() function.
*/
export default {
data: {
myArr: ['foo', 'bar', 'doo']
},
methods: {
/**
* Case 1) with myArr.forEach.
*/
snippet96Case1 () {
this.myArr.forEach(function (item, index) {
console.log(item, index)
})
},
/**
* Case 2) with ES6 for-of statement.
*/
snippet96Case2 () {
for (const [index, item] of Object.entries(this.myArr)) {
console.log(item, index)
}
},
/**
* Case 3) with ES6 for-of statement without the index.
*/
snippet96Case3 () {
for (const item of this.myArr) {
console.log(item)
}
}
}
}
More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
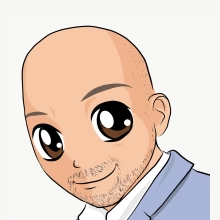