Getting a DateTime object from a DateTimeImmuntable object with PHP
Published on 2023-01-03 • Modified on 2023-01-03
This snippet shows how to get a DateTime object from a DateTimeImmuntable object with PHP. We can use the setTimestamp()
function or the createFromImmutable
static function, which is more elegant, IMHO.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet239Trait
{
public function snippet239(): void
{
$date = '2023-01-03';
// 1. with setTimeStamp
$immutable = new \DateTimeImmutable($date);
$mutable = (new \DateTime())->setTimestamp($immutable->getTimestamp());
echo $mutable->format(\DateTimeInterface::ATOM).PHP_EOL;
// 2. using createFromImmutable
$mutable = \DateTime::createFromImmutable($immutable);
echo $mutable->format(\DateTimeInterface::ATOM).PHP_EOL;
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
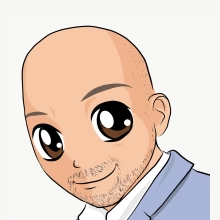