Creating a fixed-size array with PHP
Published on 2022-11-11 • Modified on 2022-11-11
This snippet shows how to create a fixed-size array with PHP. We can use the SplFixedArray
class. It is interesting to use this structure sometimes for large arrays as it is optimized in memory. Note that we could expect a OutOfBoundsException
if we try to access an invalid index, but we get a RuntimeException
instead.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet231Trait
{
public function snippet231(): void
{
$splFixedArray = new \SplFixedArray(5);
$splFixedArray[3] = 'foo';
$splFixedArray[4] = 'bar';
var_dump($splFixedArray);
echo 'Size of array: '.$splFixedArray->count().PHP_EOL.PHP_EOL;
// out of range index
try {
$splFixedArray[10] = 'oops!!';
} catch (\RuntimeException $e) {
echo 'Error: '.$e->getMessage().PHP_EOL.PHP_EOL;
}
var_dump($splFixedArray->toArray());
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
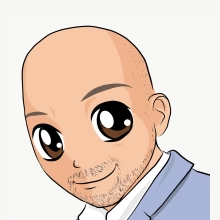