Check if the Symfony debug mode is activated
Published on 2019-07-22 • Modified on 2019-07-22
Sometimes it's useful to know if the debug mode is activated. (with the famous debug bar) For example for a batch process, if it is on, perhaps you only want to process some rows instead of processing the whole table. To get the flag you need to get the kernel instance and call the isDebug
function.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Repository\ArticleRepository;
use Symfony\Component\HttpKernel\KernelInterface;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property ArticleRepository $articleRepo
* @property KernelInterface $kernel
*/
trait Snippet33Trait
{
public function snippet33(): void
{
echo $this->kernel->isDebug() ? 'Debug is ✅' : 'Debug is ❌';
$maxToProcess = $this->kernel->isDebug() ? 2 : null; // null in this context means to maximum
$snippets = $this->articleRepo->findSnippetsForLang('en', null, $maxToProcess);
echo PHP_EOL.\sprintf('%d snippets to process! 🏭', \count($snippets));
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet33Trait
*/
final class Snippet33Test extends KernelTestCase
{
protected function setUp(): void
{
self::bootKernel();
}
/**
* @see Snippet33Trait::snippet33
*/
public function testSnippet33(): void
{
self::assertTrue(self::$kernel->isDebug());
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
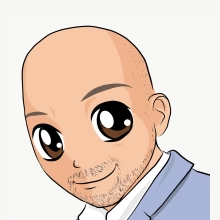