Add a get parameter to the current URL with JavaScript
Published on 2019-02-27 • Modified on 2019-02-27
Here is a clean way to do this using the URL JavaScript object. In this snippet, 1) We get the current URL 2) We create an URL object with the current url we've just retrieved. 3) we apprend and set get parameters to it. 4) Then, we change the current url of the browser without reloading the page. Et voilà! 😉
PS: Note that if you hit the refresh button of your browser, the id parameter will be append another time, not the foo parameter because we use the set function that prevents it from being added more than one time. (so most of the time we just need the set function)
/**
* I am using a JavaScript module to isolate the code of each snippet.
* In fact it's a Vue.js mixin. Take the code called by the mounted()
* or the snippetXX() function.
*/
export default {
data: {
id: 17
},
methods: {
snippet17 () {
const urlStr = window.location.href
const urlObj = new URL(urlStr)
urlObj.searchParams.append('foo', 'bar')
urlObj.searchParams.append('id', this.id)
urlObj.searchParams.set('foo', 'bar2')
history.replaceState({}, '', urlObj.toString())
console.log('Refresh the page and check the URL! 🧐')
}
},
mounted () {
if (this.isArticle(this.id)) {
this.snippet17()
}
}
}
More on Stackoverflow Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
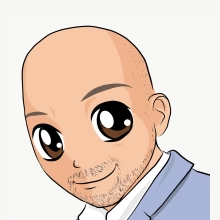