Récupérer le premier caractère d'une chaîne avec PHP
Publié le 12/11/2021 • Actualisé le 12/11/2021
Dans ce bout de code, nous voyons comment récupérer le premier caractère d'une chaîne avec PHP et Symfony. Dans la première partie, nous voyons comment utiliser la notation par accès tableau. On doit être vigilant, car ça ne marche pas avec les caractères spéciaux. Dans ce cas, on doit utiliser la fonction mb_substr
. Dans la deuxième partie, nous voyons comment utiliser le composant String de Symfony. Il gère automatiquement les caractères spéciaux, les chaînes vides et les valeurs nulles.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Helper\String\StringHelper;
use function Symfony\Component\String\u;
/**
* J'utilise un trait PHP afin d'isoler chaque snippet dans un fichier.
* Ce code doit être apellé d'un contrôleur Symfony étendant AbstractController (depuis Symfony 4.2)
* ou Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Les services sont injectés dans le constructeur du contrôleur principal.
*
* @property StringHelper $stringHelper
*/
trait Snippet173Trait
{
public function snippet173(): void
{
echo "—— Array access ——————————————————————————————————————————————\n";
$str = 'ABCD';
echo 'Case n°1 : ';
echo $str[0]; // A
echo "\n";
$str = 'äBCD';
echo 'Case n°2 : ';
echo $str[0]; // � => Doesn't work because ä is a special character
echo "\n";
echo 'Case n°3 : ';
echo mb_substr($str, 0, 1); // ä => OK using mb_substr with a special character
echo "\n";
$str = '';
echo 'Case n°4 : ';
echo @$str[0]; // PHP Warning. PHPStan error: Offset 0 does not exist on ''. @phpstan-ignore-line
echo "\n";
$str = null;
echo 'Case n°5 : ';
echo @$str[0]; // PHP Warning. PHPStan error: Offset 0 does not exist on null. @phpstan-ignore-line
echo "\n";
echo "\n";
echo "—— With the Symfony string component —————————————————————————\n";
$str = u('ABCD');
echo 'Case n°6 : ';
echo $str->slice(0, 1); // A
echo "\n";
$str = u('äBCD');
echo 'Case n°7 : ';
echo $str->slice(0, 1); // ä => The Symfony component automatically hanldes special characters
echo "\n";
$str = u('');
echo 'Case n°8 : ';
echo $str->slice(0, 1); // "" => returns an empty string in this case
echo "\n";
$str = u(null);
echo 'Case n°9 : ';
echo $str->slice(0, 1); // "" => also returns an empty string in this case
echo "\n\n";
echo "—— With my Symfony string component helper ———————————————————\n";
echo 'Case n°10 : ';
echo $this->stringHelper->u('ABCD')->firstChar(); // "A"
echo "\n";
echo 'Case n°11 : ';
echo $this->stringHelper->u('äBCD')->firstChar(); // "ä"
echo "\n";
echo 'Case n°12 : ';
echo $this->stringHelper->u('')->firstChar(); // ""
echo "\n";
echo 'Case n°13 : ';
echo $this->stringHelper->u(null)->firstChar(); // ""
// this firstChar() function is a custom function I have added (blog post to come)
// That's it! 😁
}
}
Exécuter le snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ Plus sur Stackoverflow Lire la doc Plus sur le web
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
use function Symfony\Component\String\u;
/**
* @see Snippet173Trait
*/
final class Snippet173Test extends KernelTestCase
{
/**
* @see Snippet173Trait::snippet173
*/
public function testSnippet173(): void
{
$str = 'ABCD';
self::assertSame('A', $str[0]);
$str = 'äBCD';
self::assertNotSame('ä', $str[0]);
$str = 'äBCD';
self::assertSame('ä', mb_substr($str, 0, 1));
$str = u('ABCD');
self::assertSame('A', $str->slice(0, 1)->toString());
$str = u('äBCD');
self::assertSame('ä', $str->slice(0, 1)->toString());
$str = u('');
self::assertSame('', $str->slice(0, 1)->toString());
$str = u(null);
self::assertSame('', $str->slice(0, 1)->toString());
}
}
A vous de jouer !
Ces articles vous ont été utiles ? Vous pouvez m'aider à votre tour de plusieurs manières : (cliquez sur le lien "reply" dans à droite pour me contacter )
- Me remonter des erreurs ou typos.
- Me remonter des choses qui pourraient être améliorées.
- Aimez et repostez !
- Suivez moi sur Bluesky 🦋
- Inscrivez-vous au flux RSS.
- Cliquez sur les boutons Plus sur Stackoverflow pour me faire gagner des badges "annonceur" 🏅.
Merci et à très bientôt sur Strangebuzz ! 😉
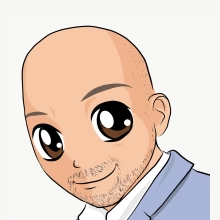