[PHP] Modifier les valeurs d'un tableau avec array_map et une fonction anonyme
Publié le 03/11/2019 • Actualisé le 03/11/2019
Voilà comment modifier toutes les valeurs d'un tableau PHP avec une fonction anonyme (🇬🇧 closure). Veuillez noter que quand on utilise array_map
, la closure ne connait pas la clé associée à la valeur qu'elle est en train de traiter. Si vous avez besoin de la clé, utilisez array_walk
.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* J'utilise un trait PHP afin d'isoler chaque snippet dans un fichier.
* Ce code doit être apellé d'un contrôleur Symfony étendant AbstractController (depuis Symfony 4.2)
* ou Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Les services sont injectés dans le constructeur du contrôleur principal.
*/
trait Snippet50Trait
{
public function snippet50(): void
{
$array = [
'kikoo' => 'cat',
'foo' => 'bar',
];
$array1 = array_map(static fn ($value) => $value.$value, $array); // with an arrow function as of PHP 7.4
// the old way before PHP 7.4
// $array1 = array_map(static function ($value): string {
// return $value.$value;
// }, $array);
// for simple cases, pass the PHP function name directly
$array2 = array_map('strtoupper', $array);
print_r($array1);
print_r($array2);
// That's it! 😁
}
}
Exécuter le snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ Plus sur Stackoverflow Lire la doc Lancer sur 3v4l.org
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet50Trait
*/
final class Snippet50Test extends KernelTestCase
{
/**
* @see Snippet50Trait::snippet50
*/
public function testSnippet50(): void
{
$array = [
'kikoo' => 'cat',
'foo' => 'bar',
];
$array1 = array_map(static function ($value): string {
return $value.$value;
}, $array);
self::assertSame([
'kikoo' => 'catcat',
'foo' => 'barbar',
], $array1);
$array2 = array_map('strtoupper', $array);
self::assertSame([
'kikoo' => 'CAT',
'foo' => 'BAR',
], $array2);
}
}
A vous de jouer !
Ces articles vous ont été utiles ? Vous pouvez m'aider à votre tour de plusieurs manières : (cliquez sur le lien "reply" dans à droite pour me contacter )
- Me remonter des erreurs ou typos.
- Me remonter des choses qui pourraient être améliorées.
- Aimez et repostez !
- Suivez moi sur Bluesky 🦋
- Inscrivez-vous au flux RSS.
- Cliquez sur les boutons Plus sur Stackoverflow pour me faire gagner des badges "annonceur" 🏅.
Merci et à très bientôt sur Strangebuzz ! 😉
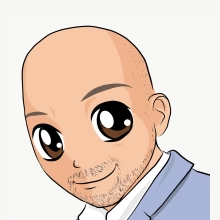