Using a high order function to filter a JavaScript array
Published on 2019-11-15 • Modified on 2019-11-15
This is an example of modern usage of JavaScript and functional programming you must know to produce concise and readable code. In this case, we filter an array on one of the properties of the objects it contains. Then, we display the labels of the filtered array. The goal is to avoid using loops and creating new functions.
/**
* I am using a JavaScript module to isolate the code of each snippet.
* In fact it's a Vue.js mixin. Take the code called by the mounted()
* or the snippetXX() function.
*/
export default {
methods: {
snippet55 () {
const todos = [
{ label: 'Call mum', type: 'todo', isUrgent: true },
{ label: 'Buy food', type: 'tobuy', isUrgent: false },
{ label: 'Write a new snippet', type: 'todo', isUrgent: true }
]
console.log(todos)
const isUrgent = todo => todo.isUrgent
const urgents = todos.filter(isUrgent)
console.log('Urgent todos are: ' + urgents.map(todo => todo.label).join(', ')) // That's it! 😁
}
},
mounted () {
if (this.isArticle(55)) {
this.snippet55()
}
}
}
More on Stackoverflow More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
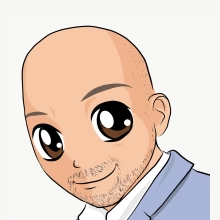