The PHP interfaces' cheatsheet
Published on 2021-06-15 • Modified on 2021-06-15
In this snippet, we see a typical PHP interface. I have added comments inside about what is allowed are not. For each case, I have added the corresponding error message. This is something every PHP developer should know! The PHP version reference for this snippet is 7.4.
<?php
declare(strict_types=1);
namespace App\Samples;
// use App\Controller\PaginationTrait; // cannot use traits inside interfaces ❌
use Symfony\Component\HttpKernel\HttpKernelInterface;
/**
* The PHP interfaces cheatsheet: PHP 7.4.
*/
interface MyHttpKernelInterface extends HttpKernelInterface // interfaces can extend other interfaces ✅
{
// use PaginationTrait; // cannot use traits inside interfaces ❌
/**
* Error= "Compile Error: Cannot use traits inside of interfaces. PaginationTrait is used in App\Samples\MyHttpKernelInterface".
*/
public const MY_CONST = '2'; // constants are allowed ✅
// public const MAIN_REQUEST = false; // Throws an error if the constant is alteady delcared in the parent interface:
/**
* Error: "Compile Error: Cannot inherit previously-inherited or override constant MAIN_REQUEST from interface Symfony\Component\HttpKernel\HttpKernelInterface".
*/
// public static $toto = 'foo'; // public static member not allowed ❌
/**
* Error : Compile Error: Interfaces may not include member variables.
*/
// protected function writePrivateProtected(string $key, string $content): int; // private / protected method declaration NOT allowed ❌
/**
* Error: "Compile Error: Access type for interface method App\Samples\MyHttpKernelInterface::writePrivateProtected() must be omitted".
*/
// public function handle(); // cannot change the signature of a method of an extended interface ❌
/**
* Error: "Compile Error:
* Declaration of App\Samples\MyHttpKernelInterface::handle($message, array $context = Array, int $myIntrefaceParam = 2)
* must be compatible with
* Symfony\Component\HttpKernel\HttpKernelInterface::handle(Symfony\Component\HttpFoundation\Request $request, int $type = self::MAIN_REQUEST, bool $catch = true).
*/
public function write(string $key, string $content): int; // public method declaration allowed ✅
public function exists(string $key): bool; // ✅
// Interfaces cannot have body ❌
/*
public function bodyNotAllowed(string $key)
{
}
*/
/*
* Error: "Compile Error: Interface function App\Samples\MyHttpKernelInterface::bodyNotAllowed() cannot contain body"
*/
}
More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
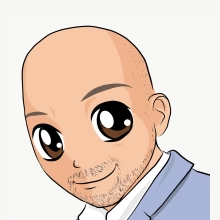