Testing the current PHP version
Published on 2021-11-19 • Modified on 2021-11-19
In this snippet, we see how to test the current PHP version. The most straightforward way is to use the PHP_VERSION_ID
constant. It's accessible to understand: The first digit is for the major version 8
. The following two numbers are for the minor version, and the last ones are for the release version. 80100
means 8.1
, 80012
means 8.0.12
and so on. For example, in the following unit test, I check if I use a version below PHP 8.1
. If it is the case, I run specific tests which would fail with PHP 8.1
as private properties are now accessible through reflection. I have to do this because my CI runs the project on both PHP 8.0
and 8.1
to ensure my project is ready for this next minor version.
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
use Symfony\Component\HttpFoundation\Request;
/**
* @see Snippet74Trait
*/
final class Snippet61Test extends KernelTestCase
{
/**
* @see Snippet61Trait::snippet61
*/
public function testSnippet61(): void
{
$request = Request::create('/en/snippets/modifying-a-private-class-property-at-runtime');
$property = (new \ReflectionClass($request))->getProperty('isHostValid');
// As of PHP 8.1, private properties are accessible with reflection!
// @see https://phpbackend.com/blog/post/php-8-1-accessing-private-protected-properties-methods-via-reflection-api-is-now-allowed-without-calling-setAccessible
if (PHP_VERSION_ID < 80100) {
try {
self::assertNull($property->getValue($request));
self::fail('A ReflectionException should be raised!');
} /* @noinspection PhpRedundantCatchClauseInspection */
catch (\ReflectionException) {
}
}
$property->setAccessible(true);
self::assertTrue($property->getValue($request));
$property->setValue($request, false);
self::assertFalse($property->getValue($request));
}
}
More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
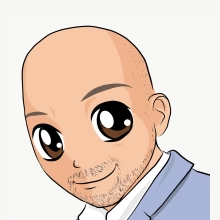