Testing if a variable is defined with JavaScript
Published on 2020-06-04 • Modified on 2020-06-04
In this snippet, we will see how to test if a variable is defined with JavaScript. To correctly understand the following code, we must make the difference between "declared" and "defined". A variable can be declared but undefined. That what shows the second case. The first case will raise an error as we are trying to use a variable that it isn't declared yet. In the third case, we check if a variable is declared using the in window
statement. In the fourth case, we use typeof
to check if the variable isn't declared or is undefined. Eventually, in the fifth case, we check that we have the same result as the fourth case, even the variable is declared. Open your browser's JavaScript console and click on the buttons to check the results. Note, that I add to disable eslint for the first case code or my GitHub actions build would have been broken. So, yes, static analysers are useful! š
/**
* I am using a JavaScript module to isolate the code of each snippet.
* In fact it's a Vue.js mixin. Take the code called by the mounted()
* or the snippetXX() function.
*/
export default {
methods: {
/**
* Case 1) foo is not declared
* This code throws "ReferenceError: foo is not defined"
*/
snippet94Case1 () {
// eslint-disable-next-line
if (foo === undefined) {
}
},
/**
* Case 2) foo is declared, test with undefined
*/
snippet94Case2 () {
let foo
if (foo === undefined) {
console.log('Case 2: foo is declared but undefined.')
}
},
/**
* Case 3) foo is not declared, test with "in" on the window object
*/
snippet94Case3 () {
if ('foo' in window) {
console.log("We don't go here as foo wasn't declared.")
} else {
console.log('Case 3: foo is not declared, no error with the "in window" test.')
}
},
/**
* Case 4) foo is not declared, test with "typeof".
*/
snippet94Case4 () {
if (typeof foo === 'undefined') {
console.log('Case 4: foo is not declared, test with "typeof".')
}
},
/**
* Case 5) foo is declared, test with "typeof".
*/
snippet94Case5 () {
let foo
if (typeof foo === 'undefined') {
console.log('Case 5: foo is declared but it is undefined.')
}
}
}
}
More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky š¦
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges š .
Thank you for reading! And see you soon on Strangebuzz! š
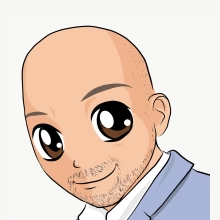