Testing if a Doctrine entity is scheduled for deletion
Published on 2022-01-12 • Modified on 2022-01-12
In this snippet, we see how to test if a Doctrine entity is scheduled for deletion. There are two different methods involving the unit of work
object. We can test a given entity, or retrieve a list of entities thanks to the getScheduledEntityDeletions()
function.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Data\ArticleData;
use App\Entity\Article;
use Doctrine\ORM\EntityManagerInterface;
use Doctrine\ORM\UnitOfWork;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property ArticleData $articleData
* @property EntityManagerInterface $entityManager
*/
trait Snippet184Trait
{
public function snippet184(): void
{
// Get the current snippet
$snippet = $this->articleData->getSnippetById(184);
// Remove it
$this->entityManager->remove($snippet);
$uow = $this->entityManager->getUnitOfWork();
if ($uow->getEntityState($snippet) === UnitOfWork::STATE_REMOVED) {
echo 'The snippet is scheduled for deletion ! ✅'.PHP_EOL;
} else {
echo 'The snippet is NOT scheduled for deletion. ❌'.PHP_EOL;
}
foreach ($uow->getScheduledEntityDeletions() as $entity) {
/** @var Article $entity */
echo \sprintf('The snippet "%s" is scheduled for deletion. ✅', $entity->getId());
}
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use App\Data\ArticleData;
use Doctrine\ORM\EntityManagerInterface;
use Doctrine\ORM\UnitOfWork;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet184Trait
*/
final class Snippet184Test extends KernelTestCase
{
private EntityManagerInterface $doctrine;
private ArticleData $articleData;
protected function setUp(): void
{
$this->doctrine = self::getContainer()->get(EntityManagerInterface::class);
$this->articleData = self::getContainer()->get(ArticleData::class);
}
/**
* @see Snippet184Trait::snippet184
*/
public function testSnippet184(): void
{
$uow = $this->doctrine->getUnitOfWork();
$snippet = $this->articleData->getArticleById(183);
$uow->remove($snippet);
self::assertSame(UnitOfWork::STATE_REMOVED, $uow->getEntityState($snippet));
self::assertCount(1, $uow->getScheduledEntityDeletions());
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
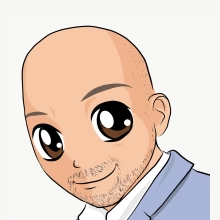