Retrieving the list of controllers of a Symfony application
Published on 2024-09-14 • Modified on 2024-09-14
This snippet shows how to retrieve the list of controllers' FQNC of a Symfony application. We can use this handy getAliases()
function on the route collection object instead of looping on all routes. It returns all combinations of controllers and actions, but if you strictly use the ADR pattern it returns the list of controllers. When using the ADR pattern, you can trim the '::__invoke
suffix of each entry. Check out the "more on the web" link to see a concrete use case of this snippet in the MicroSymfony project codebase.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Controller\BlogController;
use function Symfony\Component\String\u;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet318Trait
{
public function snippet318(): void
{
$controllers = array_unique(array_map(
static fn ($value) => u($value)->trimSuffix('::__invoke')->toString(),
array_keys($this->router->getRouteCollection()->getAliases())
));
// filter on a restricted set
$controllers = array_filter($controllers, static fn ($value) => u($value)->startsWith(BlogController::class));
var_dump($controllers);
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
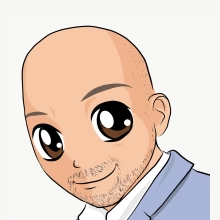