Ordering a PHP array of objects by a given property
Published on 2023-05-31 • Modified on 2023-05-31
This snippet shows how to order a PHP array of objects by a given property. The sort function is in the snippet class, but if we want to put the comparison function inside the object class, we can use a static function using the new first-class callable syntax introduced in PHP 8.1.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Samples\SortableObject;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet257Trait
{
public function snippet257(): void
{
$array = [];
$array[] = new SortableObject('yellow');
$array[] = new SortableObject('blue');
$array[] = new SortableObject('orange');
$array[] = new SortableObject('green');
usort($array, $this->sortSortableObject(...));
var_dump($array);
shuffle($array);
var_dump($array);
usort($array, SortableObject::sort(...));
var_dump($array);
// That's it! 😁
}
public function sortSortableObject(SortableObject $object1, SortableObject $object2): int
{
return $object1->name <=> $object2->name;
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
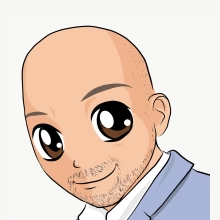