Getting the block names of a Twig template
Published on 2020-08-08 • Modified on 2020-08-08
In this snippet, we will see how to get the block names of a Twig template. To do so, we must use the Twig\Environment
service which is available in the service container. Sorry, I didn't find a doc on the subject but the GitHub PR when this function was modified.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use Twig\Environment;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property Environment $twigEnvironment
*/
trait Snippet108Trait
{
public function snippet108(): void
{
$blockNames = $this->twigEnvironment->load('layout.html.twig')->getBlockNames();
var_dump($blockNames);
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
use Twig\Environment;
/**
* @see Snippet108Trait
*/
final class Snippet108Test extends KernelTestCase
{
private Environment $twigEnvironment;
protected function setUp(): void
{
$this->twigEnvironment = self::getContainer()->get(Environment::class);
}
/**
* @see Snippet108Trait::snippet108
*/
public function testSnippet108(): void
{
$blockNames = $this->twigEnvironment->load('layout.html.twig')->getBlockNames();
self::assertCount(14, $blockNames);
}
/**
* Fake test to test tearDown() and tearDownAfterClass().
*/
public function testSnippet108Part2(): void
{
$blockNames = $this->twigEnvironment->load('layout.html.twig')->getBlockNames();
self::assertCount(14, $blockNames);
}
/**
* Executed after each test. It is protected and NOT static.
*
* @see certif/Tests/20210601.txt
*/
protected function tearDown(): void
{
// echo 'tearDown'."\n";
}
/**
* Executed after the last test. It is public AND static.
*/
public static function tearDownAfterClass(): void
{
// echo 'tearDown after class'."\n";
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
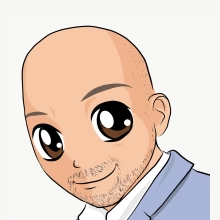