Getting the activated PHP extensions list with their versions
Published on 2021-10-07 • Modified on 2021-10-07
In this snippet, we see how to get the activated PHP extensions list with their versions. I use a one-line statement with an arrow function, but the code with a simple loop is more readable. With the terminal, one can use php -m
, but it doesn't give the version.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet168Trait
{
public function snippet168(): void
{
$extensionsWithVersion = array_combine(get_loaded_extensions(), array_map(static fn (string $extension) => phpversion($extension), get_loaded_extensions()));
var_dump($extensionsWithVersion);
// loop version
$extensionsWithVersion = [];
foreach (get_loaded_extensions() as $extension) {
$extensionsWithVersion[$extension] = phpversion($extension);
}
var_dump($extensionsWithVersion);
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc More on the web
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet168Trait
*/
final class Snippet168Test extends KernelTestCase
{
/**
* @see Snippet168Trait::snippet168
*/
public function testSnippet168(): void
{
$extensionsWithVersion = array_combine(get_loaded_extensions(), array_map(static fn (string $extension) => phpversion($extension), get_loaded_extensions()));
$extensionsWithVersionLoop = [];
foreach (get_loaded_extensions() as $extension) {
$extensionsWithVersionLoop[$extension] = phpversion($extension);
}
self::assertSame($extensionsWithVersion, $extensionsWithVersionLoop);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
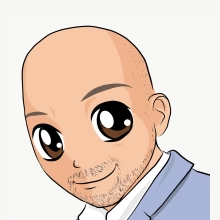