Get the Symfony and PHP versions of the current environment
Published on 2019-01-15 • Modified on 2023-01-06
It is sometimes helpful to have this information. There are various ways to get the Symfony version. In CLI, we can use the bin/console --version
command or, with composer composer info symfony/http-kernel | grep versions
. We can also see the version in the profiler bar.
On this blog, I display them to show you the environment the code is running in so you can compare them with your own. It allows us to avoid hardcode values, and all will be up to date even after system migrations or composer updates.
<?php declare(strict_types=1);
namespace App\Twig\Extension;
use Symfony\Component\HttpFoundation\RequestStack;
use Symfony\Component\HttpKernel\Kernel;
/**
* Server environment related stuff.
*/
class EnvExtension extends \Twig_Extension implements \Twig_Extension_GlobalsInterface
{
public function getGlobals(): array
{
$phpVersion = PHP_VERSION;
$parts = explode('.', $phpVersion);
$phpMinorVersion = $parts[0].'.'.$parts[1];
$phpVersion = $phpMinorVersion.'.'.explode('-', $parts[2])[0] ?? '';
$sfVersion = Kernel::VERSION;
$sfVersionInfos = explode('.', Kernel::VERSION);
$globals = [
'php_version' => $phpVersion,
'php_minor_version' => $phpMinorVersion,
'sf_version' => $sfVersion,
'sf_minor_version' => $sfVersionInfos[0].'.'.$sfVersionInfos[1],
];
return $globals;
}
}
Bonus, the snippet to run this code: 🎉<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
use App\Twig\Extension\EnvExtension;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*
* @property EnvExtension $envExtension
*/
trait Snippet12Trait
{
public function snippet12(): void
{
print_r($this->envExtension->getGlobals()); // That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use App\Twig\Extension\EnvExtension;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet12Trait
*/
final class Snippet12Test extends KernelTestCase
{
private EnvExtension $envExtension;
protected function setUp(): void
{
$this->envExtension = self::getContainer()->get(EnvExtension::class);
}
/**
* @see Snippet12Trait::snippet12
*/
public function testSnippet12(): void
{
$globals = $this->envExtension->getGlobals();
self::assertArrayHasKey('php_version', $globals);
self::assertArrayHasKey('php_minor_version', $globals);
self::assertArrayHasKey('sf_version', $globals);
self::assertArrayHasKey('sf_minor_version', $globals);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
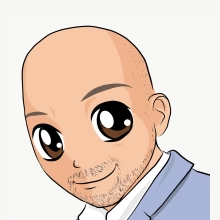