[Symfony] Creating an event subscriber for a 404 NotFoundHttpException
Published on 2023-03-30 • Modified on 2023-03-30
This snippet shows how to create an event subscriber for a 404 NotFoundHttpException
. Here, we test if a given path was accessed (this path doesn't math any application route). We can also log something or notify; check out the documentation example. You can test the snippet here.
<?php
declare(strict_types=1);
namespace App\Subscriber;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\HttpKernel\Event\ExceptionEvent;
use Symfony\Component\HttpKernel\Exception\NotFoundHttpException;
final class NotFoundExceptionSubscriber implements EventSubscriberInterface
{
public static function getSubscribedEvents(): array
{
return [
ExceptionEvent::class => 'onKernelException',
];
}
public function onKernelException(ExceptionEvent $event): void
{
$exception = $event->getThrowable();
if ($exception instanceof NotFoundHttpException) {
$path = $event->getRequest()->getPathInfo();
if ($path === '/404') {
$event->setResponse(new Response('404 found!'));
$event->stopPropagation();
}
}
}
}
More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
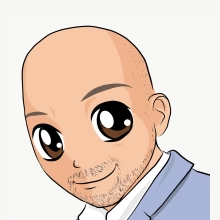