Creating a two dimensions array with PHP
Published on 2021-12-30 • Modified on 2021-12-30
In this snippet, we see how to create a two dimensions array with PHP. Of course, we can make loops with the range()
function but we use a one-liner here with array_fill()
. In this case, we have a "square"; if you want another size, the first count is the height while the second one is the array width. Note that the snippet on the PHP website is 17 years old as I write theses lines!
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet181Trait
{
public function snippet181(): void
{
$array = array_fill(1, 4, array_fill(1, 4, ''));
var_dump($array);
// That's it! 😁
}
}
Run this snippet ≪ this.showUnitTest ? this.trans.hide_unit_test : this.trans.show_unit_test ≫ More on Stackoverflow Read the doc Random snippet
<?php
declare(strict_types=1);
namespace App\Tests\Integration\Controller\Snippets;
use Symfony\Bundle\FrameworkBundle\Test\KernelTestCase;
/**
* @see Snippet181Trait
*/
final class Snippet181Test extends KernelTestCase
{
/**
* @see Snippet181Trait::snippet181
*/
public function testSnippet181(): void
{
$array = array_fill(1, 4, array_fill(1, 4, ''));
self::assertCount(4, $array);
self::assertCount(4, $array[1]);
self::assertCount(4, $array[2]);
self::assertCount(4, $array[3]);
self::assertCount(4, $array[4]);
}
}
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
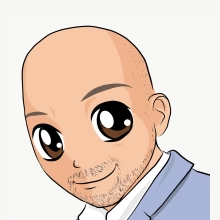