Converting a GET parameter to a bool type with PHP
Published on 2023-09-01 • Modified on 2023-09-01
This snippet shows how to convert a GET parameter to a bool type with PHP. Sometimes, when you need to convert a GET filter on an API endpoint, you need to transform this parameter into a boolean variable: true
or false
. You could use a bool cast, but this can have unexpected results. For example, (bool) "false"
, returns true
, not false
. To address this problem, we can use the filter_var
function with the FILTER_VALIDATE_BOOLEAN
flag.
<?php
declare(strict_types=1);
// src/Controller/Snippet/Snippet268Trait.php
namespace App\Controller\Snippet;
use Symfony\Component\HttpFoundation\Request;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet268Trait
{
public function snippet268(Request $request): void
{
$values = [
// true
'on',
'true',
'1',
'yes',
1,
true,
// false
'off',
'false',
'0',
'no',
'foo',
[],
0,
false,
null,
];
foreach ($values as $value) {
var_dump($value);
var_dump(filter_var($value, FILTER_VALIDATE_BOOL));
echo PHP_EOL;
}
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
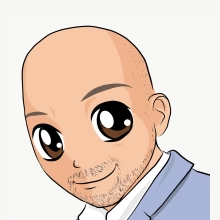