Checking if a string contains valid JSON with PHP
Published on 2024-02-07 • Modified on 2024-02-07
This snippet shows how to check if a string contains valid JSON with PHP. This function was introduced in PHP 8.3 to avoid using json_decode
or to catch an exception when using the JSON_THROW_ON_ERROR
flag. The json_last_error_msg
function still works to get the exact error message regarding the validation failure. Note that this function only accepts a string as the first argument.
<?php
declare(strict_types=1);
namespace App\Controller\Snippet;
/**
* I am using a PHP trait to isolate each snippet in a file.
* This code should be called from a Symfony controller extending AbstractController (as of Symfony 4.2)
* or Symfony\Bundle\FrameworkBundle\Controller\Controller (Symfony <= 4.1).
* Services are injected in the main controller constructor.
*/
trait Snippet289Trait
{
public function snippet289(): void
{
$fixtures = [
// Not valid
'foo',
'{foo: bar}',
"'{'foo': 'bar'}",
'{...}',
// Valid
'"foo"',
'1',
'{"foo": "bar"}',
];
foreach ($fixtures as $string) {
if (json_validate($string)) {
echo \sprintf('YES, >%s< is a valid JSON string', $string).PHP_EOL;
echo 'decoded: '.var_export(json_decode($string), true).PHP_EOL;
} else {
echo \sprintf('NO, >%s< is NOT a valid JSON string: %s', $string, json_last_error_msg()).PHP_EOL;
}
}
// That's it! 😁
}
}
Run this snippet More on Stackoverflow Read the doc More on the web Random snippet
Call to action
Did you like this post? You can help me back in several ways: (use the "reply" link on the right to comment or to contact me )
- Report any error/typo.
- Report something that could be improved.
- Like and repost!
- Follow me on Bluesky 🦋
- Subscribe to the RSS feed.
- Click on the More on Stackoverflow buttons to make me win "Announcer" badges 🏅.
Thank you for reading! And see you soon on Strangebuzz! 😉
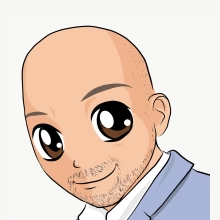